I'm using DataGrid's Custom Filter that has long load times with a RadzenDatePicker. When selecting the date via the datepicker, the Loader shows correctly.
When typing the date manually, and leaving (blur) the datepicker, the Loader is not shown.
I also tried adding isLoading = true;
as the first line in the Change event, but that did not make a difference.
--I also experienced similar issues with RadzenRequiredValidator not updating when the bind-Value property gets updated via other means than using the datepicker.
I cannot provide working code, as this table has hundreds of thousands of records with sensitive data.
Do these gifs provide enough?
On Blur:
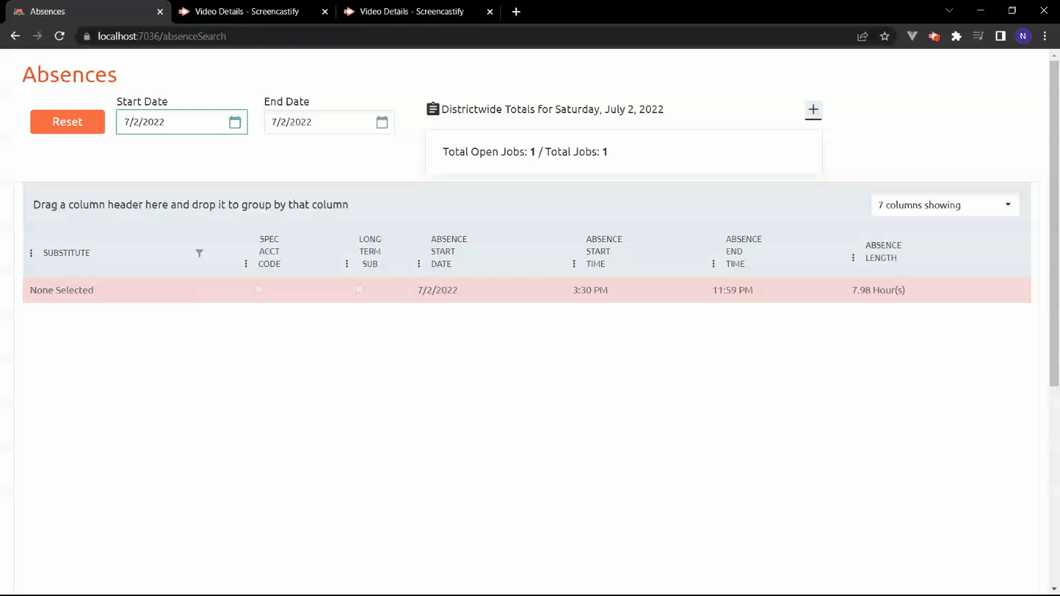
On Datepicker Select:
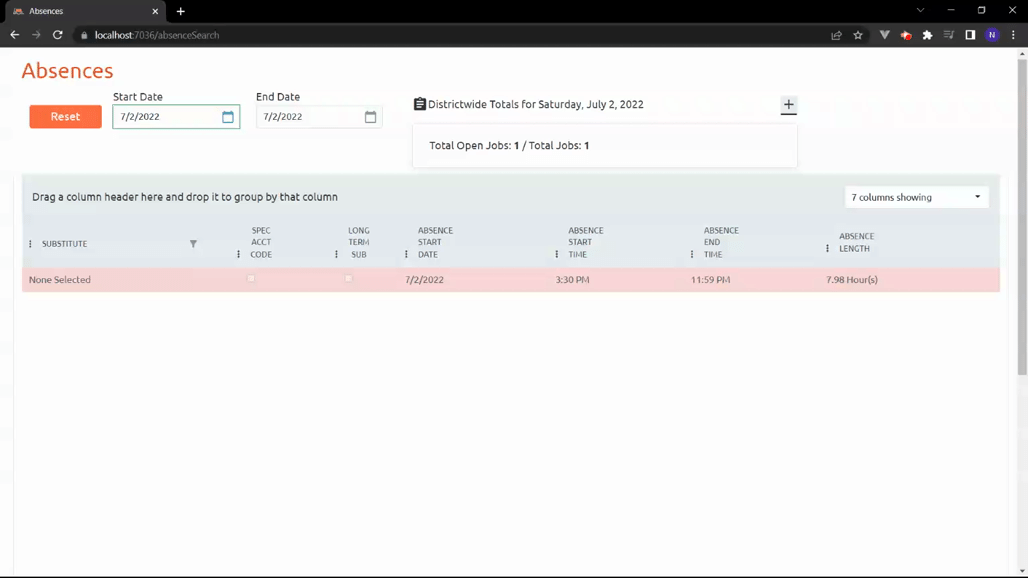
Ok I threw together a working Northwind example that will show the issue with RadzenRequiredValidator.
Steps to reproduce:
- Click Search to make form invalid
- Click Populate Dates to populate the datepickers via bound properties.
- Click Search again.
Nothing happens.
LoadData doesn't get fired again until you go to a datepicker and select a date.
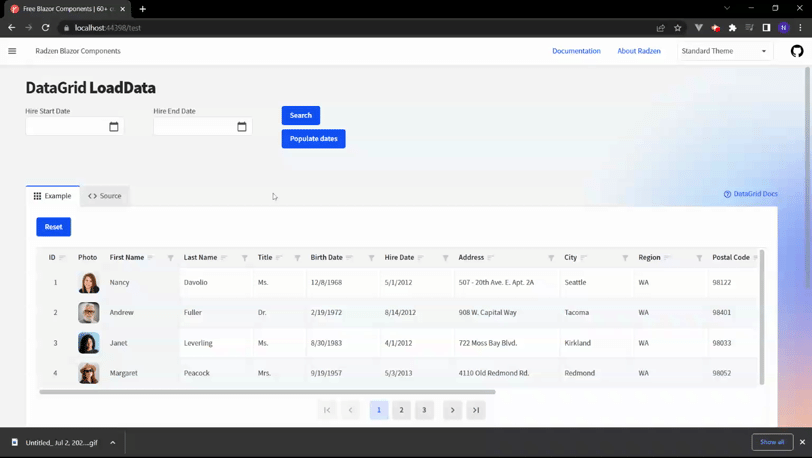
@page "/test"
@using System.Linq.Dynamic.Core
@using RadzenBlazorDemos.Data
@using RadzenBlazorDemos.Models.Northwind
@inject NorthwindContext dbContext
<h1>DataGrid <strong>LoadData</strong></h1>
<RadzenTemplateForm TItem="Employee" Data="@searchData" Style="margin-bottom: 5em;">
<div class="row mb-10">
<div class="col-xl-2">
<label for="hireStartDateSearch">Hire Start Date</label><br />
<RadzenDatePicker id="hireStartDateSearch" Name="hireStartDateSearch" @bind-Value=@hireStartDate DateFormat="d" Change="@OnHireDateChange" /><br />
<RadzenRequiredValidator Component="hireStartDateSearch" Text="Hire Start Date is required" Popup="true" />
</div>
<div class="col-xl-2">
<label for="hireEndDateSearch">Hire End Date</label><br />
<RadzenDatePicker id="hireEndDateSearch" Name="hireEndDateSearch" @bind-Value=@hireEndDate DateFormat="d" Change="@OnHireDateChange" /><br />
<RadzenRequiredValidator Component="hireEndDateSearch" Text="Hire End Date is required" Popup="true" />
</div>
<div class="col-xl-2">
<RadzenButton ButtonType="ButtonType.Submit" Text="Search" class="mb-2" /><br />
<RadzenButton Click="@PopulateDates" Text="Populate dates" />
</div>
</div>
</RadzenTemplateForm>
<RadzenExample Name="DataGrid" Source="DataGridLoadData" Heading="false">
<RadzenButton Text="Reset" Click="@Reset" Style="margin-bottom: 20px;" />
<RadzenDataGrid style="height: 335px" @ref="grid" IsLoading=@isLoading Count="@count" Data="@employees" LoadData="@LoadData" AllowSorting="true" AllowFiltering="true" AllowPaging="true" PageSize="4" PagerHorizontalAlign="HorizontalAlign.Center" TItem="Employee" ColumnWidth="200px">
<Columns>
<RadzenDataGridColumn TItem="Employee" Property="EmployeeID" Filterable="false" Title="ID" Frozen="true" Width="70px" TextAlign="TextAlign.Center" />
<RadzenDataGridColumn TItem="Employee" Title="Photo" Frozen="true" Sortable="false" Filterable="false" Width="60px" >
<Template Context="data">
<RadzenImage Path="@data.Photo" style="width: 40px; height: 40px; border-radius: 8px;" />
</Template>
</RadzenDataGridColumn>
<RadzenDataGridColumn TItem="Employee" Property="FirstName" Title="First Name" Frozen="true" Width="140px"/>
<RadzenDataGridColumn TItem="Employee" Property="LastName" Title="Last Name" Width="140px"/>
<RadzenDataGridColumn TItem="Employee" Property="TitleOfCourtesy" Title="Title" Width="100px" />
<RadzenDataGridColumn TItem="Employee" Property="BirthDate" Title="Birth Date" FormatString="{0:d}" Width="140px" />
<RadzenDataGridColumn TItem="Employee" Property="HireDate" Title="Hire Date" FormatString="{0:d}" Width="140px" />
<RadzenDataGridColumn TItem="Employee" Property="Address" Title="Address" Width="200px" />
<RadzenDataGridColumn TItem="Employee" Property="City" Title="City" Width="140px" />
<RadzenDataGridColumn TItem="Employee" Property="Region" Title="Region" Width="140px" />
<RadzenDataGridColumn TItem="Employee" Property="PostalCode" Title="Postal Code" Width="140px" />
<RadzenDataGridColumn TItem="Employee" Property="Country" Title="Country" Width="140px" />
<RadzenDataGridColumn TItem="Employee" Property="HomePhone" Title="Home Phone" Width="140px" />
<RadzenDataGridColumn TItem="Employee" Property="Extension" Title="Extension" Width="140px" />
<RadzenDataGridColumn TItem="Employee" Property="Notes" Title="Notes" Width="300px" />
</Columns>
</RadzenDataGrid>
</RadzenExample>
@code {
RadzenDataGrid<Employee> grid;
int count;
IEnumerable<Employee> employees;
bool isLoading = false;
DateTime? hireStartDate { get; set; }
DateTime? hireEndDate { get; set; }
private Employee searchData = new Employee();
private async Task OnHireDateChange()
{
grid.Reload();
}
private void PopulateDates()
{
hireStartDate = new DateTime(2012, 4, 1);
hireEndDate = new DateTime(2013, 4, 1);
}
async Task Reset()
{
hireStartDate = null;
hireEndDate = null;
grid.Reset(true);
await grid.FirstPage(true);
}
async Task LoadData(LoadDataArgs args)
{
isLoading = true;
await Task.Yield();
var query = dbContext.Employees.AsQueryable();
if (!string.IsNullOrEmpty(args.Filter))
{
query = query.Where(args.Filter);
}
if (hireStartDate != null && hireEndDate != null)
{
query = query.Where(x => x.HireDate >= hireStartDate.Value.AddSeconds(-1) && x.HireDate <= hireEndDate.Value.AddDays(1).AddSeconds(-1));
}
if (!string.IsNullOrEmpty(args.OrderBy))
{
query = query.OrderBy(args.OrderBy);
}
count = query.Count();
employees = query.Skip(args.Skip.Value).Take(args.Top.Value).ToList();
isLoading = false;
}
}
You can use use normal button type instead submit and execute grid.Reload()
on that button Click.
The provided code was just an example of the DatePicker blur not behaving the same as selecting a date.
In my real application (gif from first post), I don't have a Button that is being clicked. grid.Reload()
is already being called in the OnChange() of the DatePickers, but it still doesn't show the loader.